Let’s discuss how to create an empty DataFrame and append rows & columns to it in Pandas. There are multiple ways in which we can do this task.
Method #1: Create a complete empty DataFrame without any column name or indices and then appending columns one by one to it.
import pandas as pd
df = pd.DataFrame()
print (df)
df[ 'Name' ] = [ 'Ankit' , 'Ankita' , 'Yashvardhan' ]
df[ 'Articles' ] = [ 97 , 600 , 200 ]
df[ 'Improved' ] = [ 2200 , 75 , 100 ]
df
|
Output:
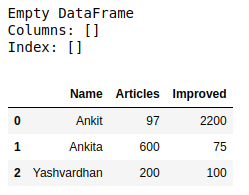
Method #2: Create an empty DataFrame with columns name only then appending rows one by one to it using append()
method.
import pandas as pd
df = pd.DataFrame(columns = [ 'Name' , 'Articles' , 'Improved' ])
print (df)
df = df.append({ 'Name' : 'Ankit' , 'Articles' : 97 , 'Improved' : 2200 },
ignore_index = True )
df = df.append({ 'Name' : 'Aishwary' , 'Articles' : 30 , 'Improved' : 50 },
ignore_index = True )
df = df.append({ 'Name' : 'yash' , 'Articles' : 17 , 'Improved' : 220 },
ignore_index = True )
df
|
Output:
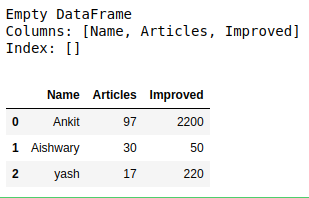
Method #3: Create an empty DataFrame with a column name and indices and then appending rows one by one to it using loc[]
method.
import pandas as pd
df = pd.DataFrame(columns = [ 'Name' , 'Articles' , 'Improved' ],
index = [ 'a' , 'b' , 'c' ])
print ( "Empty DataFrame With NaN values : \n\n" , df)
df.loc[ 'a' ] = [ 'Ankita' , 50 , 100 ]
df.loc[ 'b' ] = [ 'Ankit' , 60 , 120 ]
df.loc[ 'c' ] = [ 'Harsh' , 30 , 60 ]
df
|
Output:
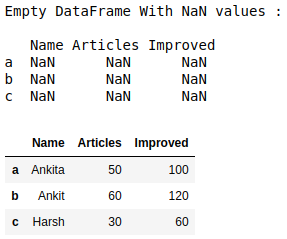
資料來源: https://www.geeksforgeeks.org/how-to-create-an-empty-dataframe-and-append-rows-columns-to-it-in-pandas/
沒有留言:
張貼留言